Colors and Background
In CSS, controlling colors and background properties is fundamental for styling web pages. This includes setting text colors, background colors, images, gradients, and more. Below are the main properties and techniques for handling colors and backgrounds in CSS.
1. Text Color
The color property sets the color of the text. Colors can be defined using various formats, such as named colors, HEX codes, RGB, RGBA, HSL, and HSLA.
Hex - Codes :
RGB (Red-Green-Blue):
HSL (Hue-Saturation-Lightness):
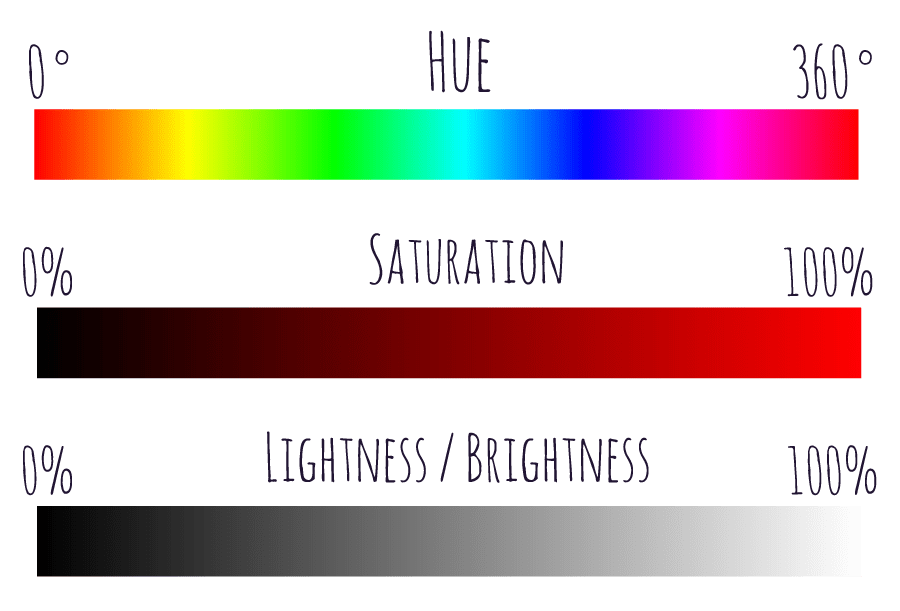
/* Named color */
p {
color: red;
}
/* HEX code */
h1 {
color: #ff5733;
}
/* RGB */
div {
color: rgb(255, 87, 51);
}
/* RGBA (with opacity) */
span {
color: rgba(255, 87, 51, 0.8);
}
/* HSL */
a {
color: hsl(11, 100%, 60%);
}
/* HSLA (with opacity) */
footer {
color: hsla(11, 100%, 60%, 0.8);
}
2. Background Color
The background-color
property sets the background color of an element.
/* Named color */
body {
background-color: lightblue;
}
/* HEX code */
header {
background-color: #333333;
}
/* RGB */
section {
background-color: rgb(240, 240, 240);
}
/* RGBA (with opacity) */
nav {
background-color: rgba(0, 0, 0, 0.5);
}
/* HSL */
article {
background-color: hsl(210, 100%, 95%);
}
/* HSLA (with opacity) */
aside {
background-color: hsla(210, 100%, 95%, 0.8);
}
3. Background Image
The background-image
property sets an image as the background of an element. You can also control its position, size, repeat behavior, and more.
/* Background image */
body {
background-image: url('path/to/image.jpg');
background-repeat: no-repeat; /* Prevents image from repeating */
background-size: cover; /* Scales image to cover the entire element */
background-position: center; /* Centers the image */
}
/* Gradient background */
div {
background-image: linear-gradient(to right, red, yellow);
}
4. Background Repeat
The background-repeat
property controls if/how a background image repeats.
div {
background-image: url('path/to/image.jpg');
background-repeat: no-repeat; /* Do not repeat the image */
background-repeat: repeat-x; /* Repeat the image horizontally */
background-repeat: repeat-y; /* Repeat the image vertically */
background-repeat: round; /* Repeat the image, but rescale to fit */
background-repeat: space; /* Repeat the image, but add space between repetitions */
}
5. Background Size
The background-size
property specifies the size of the background image.
section {
background-image: url('path/to/image.jpg');
background-size: auto; /* Default, original size */
background-size: cover; /* Scale the image to cover the element */
background-size: contain; /* Scale the image to be contained within the element */
background-size: 100px 200px; /* Specify exact size */
background-size: 50% 50%; /* Specify size as a percentage */
}
6. Background Position
The background-position
property sets the starting position of the background image.
header {
background-image: url('path/to/image.jpg');
background-position: left top; /* Align image to top left */
background-position: center; /* Center the image */
background-position: 50% 50%; /* Center the image using percentages */
background-position: 10px 20px; /* Set position using exact values */
}