Features of CodeHarborHub Documentation
Diagrams with Mermaidβ
Example Mermaid diagram
```mermaid
graph TD;
A-->B;
A-->C;
B-->D;
C-->D;
```
http://localhost:3000
Tabsβ
Example Tabs
<Tabs>
<TabItem value="apple" label="Apple" default>
This is an apple π
</TabItem>
<TabItem value="orange" label="Orange">
This is an orange π
</TabItem>
<TabItem value="banana" label="Banana">
This is a banana π
</TabItem>
</Tabs>
http://localhost:3000
- Apple
- Orange
- Banana
This is an apple π
This is an orange π
This is a banana π
Interactive code editorβ
Example Live Code Block
```jsx live
function Clock(props) {
const [date, setDate] = useState(new Date());
useEffect(() => {
const timerID = setInterval(() => tick(), 1000);
return function cleanup() {
clearInterval(timerID);
};
});
function tick() {
setDate(new Date());
}
return (
<div>
<h2>It is {date.toLocaleTimeString()}.</h2>
</div>
);
}
```
http://localhost:3000
Live Editor
function Clock(props) { const [date, setDate] = useState(new Date()); useEffect(() => { const timerID = setInterval(() => tick(), 1000); return function cleanup() { clearInterval(timerID); }; }); function tick() { setDate(new Date()); } return ( <div> <h2>It is {date.toLocaleTimeString()}.</h2> </div> ); }
Result
Loading...
Multi-language support code blocksβ
Example Multi-language code block
<Tabs>
<TabItem value="js" label="JavaScript">
```js
function helloWorld() {
console.log("Hello, world!");
}
```
</TabItem>
<TabItem value="py" label="Python">
```py
def hello_world():
print("Hello, world!")
```
</TabItem>
<TabItem value="java" label="Java">
```java
class HelloWorld {
public static void main(String args[]) {
System.out.println("Hello, World");
}
}
```
</TabItem>
<TabItem value="c" label="C">
```c
#include <stdio.h>
int main() {
printf("Hello, World\n");
return 0;
}
```
</TabItem>
</Tabs>
http://localhost:3000
- JavaScript
- Python
- Java
- C
function helloWorld() {
console.log("Hello, world!");
}
def hello_world():
print("Hello, world!")
class HelloWorld {
public static void main(String args[]) {
System.out.println("Hello, World");
}
}
#include <stdio.h>
int main() {
printf("Hello, World\n");
return 0;
}
Add Apple Style Windowβ
Example Apple Style Window
<BrowserWindow minHeight={300}>
<img src="https://github.com/Ajay-Dhangar.png" width="150" /> <br />
<button onClick={() => alert('Hello, world!')}>Click Me</button>
</BrowserWindow>
http://localhost:3000
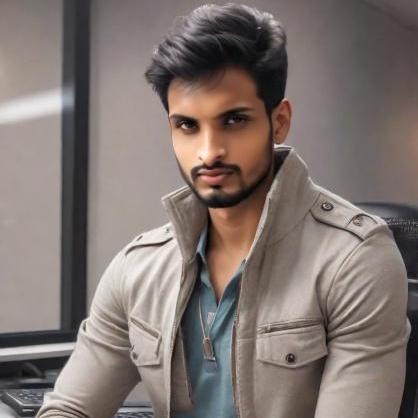
Highlighting with metadata stringβ
Example Highlighting with metadata string
```jsx {1,4-6,11}
import React from "react";
function MyComponent(props) {
if (props.isBar) {
return <div>Bar</div>;
}
return <div>Foo</div>;
}
export default MyComponent;
```
http://localhost:3000
import React from "react";
function MyComponent(props) {
if (props.isBar) {
return <div>Bar</div>;
}
return <div>Foo</div>;
}
export default MyComponent;
Line numberingβ
Example Line numbering
```jsx {1,4-6,11} showLineNumbers
import React from "react";
function MyComponent(props) {
if (props.isBar) {
return <div>Bar</div>;
}
return <div>Foo</div>;
}
export default MyComponent;
```
http://localhost:3000
import React from "react";
function MyComponent(props) {
if (props.isBar) {
return <div>Bar</div>;
}
return <div>Foo</div>;
}
export default MyComponent;
Math Equations with KaTeXβ
Math equations are rendered using KaTeX. You can write inline math equations or block equations using LaTeX.
Inlineβ
Write inline math equations by wrapping LaTeX equations between $
:
Let $f\colon[a,b]\to\R$ be Riemann integrable. Let $F\colon[a,b]\to\R$ be
$F(x)=\int_{a}^{x} f(t)\,dt$. Then $F$ is continuous, and at all $x$ such that
$f$ is continuous at $x$, $F$ is differentiable at $x$ with $F'(x)=f(x)$.
http://localhost:3000
Let be Riemann integrable. Let be . Then is continuous, and at all such that is continuous at , is differentiable at with .
Blocksβ
For equation block or display mode, use line breaks and $$
:
$$
I = \int_0^{2\pi} \sin(x)\,dx
$$
http://localhost:3000
warningβ
warning
:::warning
Beware of the dark side.
:::
http://localhost:3000
warning
Beware of the dark side.
dangerβ
danger
:::danger
I find your lack of faith disturbing.
:::
http://localhost:3000
danger
I find your lack of faith disturbing.
infoβ
info
:::info
Luke, I am your father.
:::
http://localhost:3000
info
Luke, I am your father.
successβ
success
:::success
The Force will be with you, always.
:::
http://localhost:3000
success
The Force will be with you, always.
Admonitionsβ
caution admonition
:::caution
This is a caution admonition
:::
http://localhost:3000
caution
This is a caution admonition
note admonition
:::note
This is a note admonition
:::
http://localhost:3000
note
This is a note admonition
tip admonition
:::tip
This is a tip admonition
:::
http://localhost:3000
tip
This is a tip admonition
important admonition
:::important
This is an important admonition
:::
http://localhost:3000
important
This is an important admonition